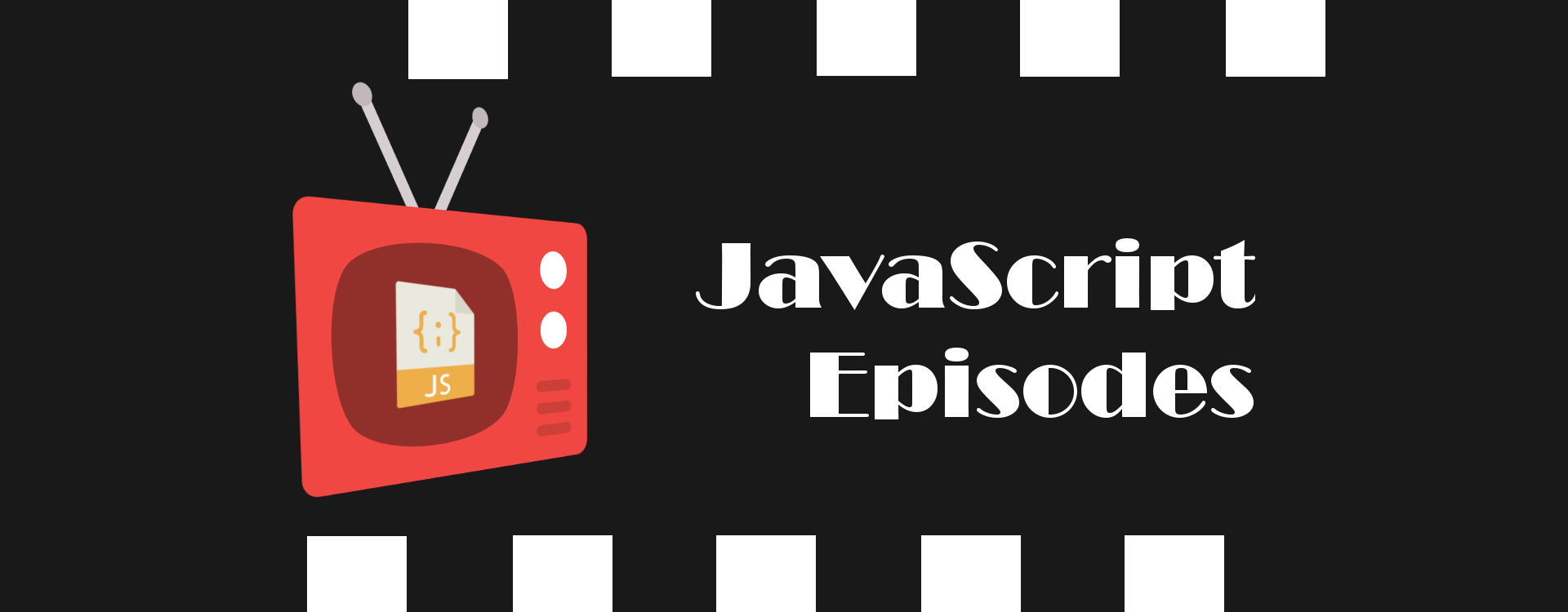
JavaScript Episodes: Ep. 1 - The Mystery of the Callback
It was Friday. A colleague of mine came up to my desk and asked me: What is a "callback"?
I made-up something on the spot:
Think about your brother borrowing your car to fill it up with gasoline and then bring it back to you, so you can go on with your trip.
But then I started really thinking about it, and came up with a better explanation. But first, let's see how a callback looks like:
function getUserInfo(userId, callback) {
fetch('/api/user-info/' + userId)
.then(response => callback(response));
}
function displayUserInfo(userInfo) {
console.log(userInfo.name);
}
getUserInfo('00132', userInfo => displayUserInfo(userInfo));
Now let's put this aside for a moment. Imagine the following scenario:
You call your brother asking him to pick up your credit card from the bank (assume that's even possible).
You'll need the info on the card to set-up your Netflix account.
So you'll ask your brother to call you back with the info when he's done.
Putting aside the security implications, how does the above scenario look when written in code?
function haveBrotherGetCreditCardInformation(accountNumber, accountSecret, callback) {
fetch('bank-which-hands-out-credit-cards-to-relatives.com/api/' + accountNumber + '/' + accountSecret)
.then(creditCardInfo => callback(creditCardInfo));
}
function setupNeflixAccount(creditCardInfo) {
fetch('netflix.com/api/create-account/', {
method: 'POST',
body: creditCardInfo
});
}
haveBrotherGetCreditCardInformation('00132', '1234', creditCardInfo => setupNeflixAccount(creditCardInfo));
So let's break it down:
- your brother will perform an asynchronous operation, that you do not know when it ends - this is the API call to the bank
- he'll call you back when he has the info on the number you gave him - this is the callback you'll send as a parameter when you ask your brother for the favor.
- You get the call back (callback) with the info you requested, received as a parameter (creditCardInfo), and you'll use it to do another action - set-up your Neflix account.
Feel free to use this explanation when someone asks you what is a callback (if you think it's any good).