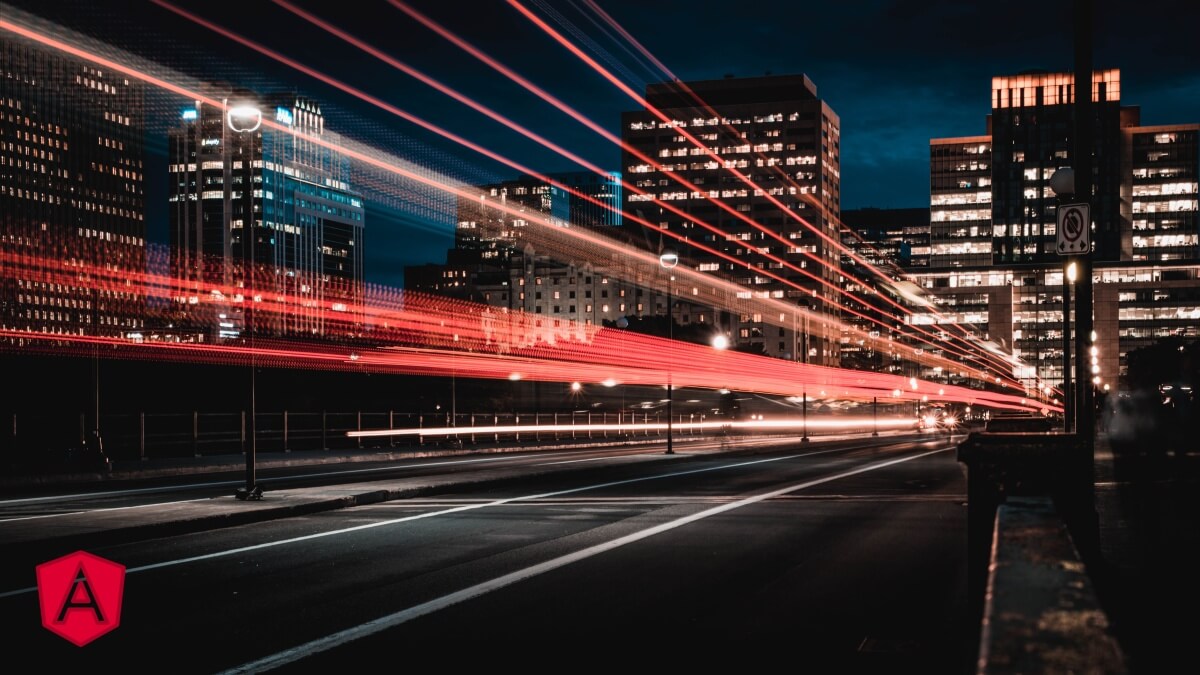
Building Performance First Angular Apps
Have you ever said "I'll just check for performance later", or "It's not really fast, but we can fix that later"?
You might not get the time for that. The best apps are built with performance in mind. I like to call them performance-first apps.
With that in mind - let's see some tips on Angular performance on HTML templates
Using pipes vs. methods
Methods are re-evaluated on each Angular change detection cycle. Pipes are pure by default, and only triggered if the input changes.
Methods in HTML - See it in action
<ul>
<li *ngFor="let user of users">
{{camelize(user.name)}}
</li>
</ul>
Pipes in HTML - See it in action
<ul>
<li *ngFor="let user of users">
{{user.name | camelize}}
</li>
</ul>
Methods vs Properties
For state properties that don't change often, use a property/variable instead of a method.
Methods in HTML
<ul *ngIf="isLoggedIn()">
<li *ngFor="let user of users">
{{ user.name }}
</li>
</ul>
Properties in HTML
<ul *ngIf="isLoggedIn">
<li *ngFor="let user of users">
{{ user.name }}
</li>
</ul>
Adding computed properties to the objects
You can add computed properties to models by determining their values in a service, and having an interface for the final model.
Computed on the fly - See it in action
<ul>
<li *ngFor="let user of users">
{{user.name}}, check: {{checksum(user.name)}}
</li>
</ul>
Pre-computed - See it in action
<ul>
<li *ngFor="let user of users">
{{user.name}}, check: {{user.checksum}}
</li>
</ul>
Don't abuse dynamic IDs
Dynamic IDs might be useful for lists, but use them wisely.
Ineffective example
<li *ngFor="let user of users; let i = index">
<span id="user-{{i}}-name">{{user.name}}</span>
<span id="user-{{i}}-age">{{user.age}}</span>
<span id="user-{{i}}-salary">{{user.salary}}</span>
</li>
Effective example
<li id="user-{{i}}" *ngFor="let user of users; let i = index">
<span class="name">{{user.name}}</span>
<span class="age">{{user.age}}</span>
<span class="salary">{{user.salary}}</span>
</li>
Use Track By
You can use track by to avoid unnecessary re-renders of the objects (if they didn't change). Read more here
Without trackBy - See it in action
<li *ngFor="let user of users">
{{user.name}}
</li>
With trackBy - See it in action
<li *ngFor="let user of users; trackBy trackByName">
{{user.name}}
</li>
Part 2 is out! Click here for more tips on fine-tuning your architecture for the best Angular Performance.
These tips & tricks don't represent an exhaustive list, and other improvements are definitely out there.
Let's chat on Twitter about these & other tips on Angular performance.